setRelationNtoN
setRelationNtoN(
string $fieldName,
string $junctionTable,
string $referrerTable,
string $primaryKeyJunctionToCurrent,
string $primaryKeyToReferrerTable,
string $referrerTitleField
[, string $sortingFieldName [, array|string $where, [, string $orderingFieldName]]]
)
At relational databases it is very common to have many to many relationship (also known as n:n or m:n). Grocery CRUD is doing it easy for you to connect 3 tables and also use it in your datagrid and forms. The syntax is easy and you just need to add the tables and the relations. All the primary keys are automatically added so you will not need to.
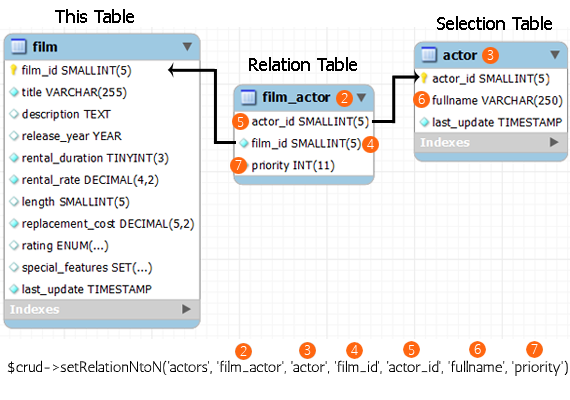
For example:
$crud->setRelationNtoN('actors', 'film_actor', 'actor', 'film_id', 'actor_id', 'fullname');
$crud->setRelationNtoN('categories', 'film_category', 'category', 'film_id', 'category_id', 'name');
$referrerTitleField
The variable $referrerTitleField
can also be written with brackets. With this way you can achieve a result to get more than one fields from the title table or create your own type of title. An example in order to understand it better is as follows:
$crud->setRelationNtoN('actors', 'film_actor', 'actor', 'film_id', 'actor_id', '{reference_id} - {first_name} {last_name}');
The above example will output strings that will take the fields from actor.reference_id
, actor.first_name
and actor.last_name
. The final output will look like this "345 - John Smith"
, "367 - George Brown"
,... e.t.c.
$where
The where statement will take the exact same format as where function of grocery CRUD Enterprise.
For example a common usage will look like this:
$crud->setRelationNtoN('actors', 'film_actor', 'actor', 'film_id', 'actor_id', 'fullname', null, [
'actor.state' => 'public'
]);
$sortingFieldName
The sorting field name is the field that you want to sort the results if you have a field in the database at the juction table. You can drag and drop the sorting field in order to sort the results.
For example:
$crud->setRelationNtoN(
'actors', 'film_actor',
'actor', 'film_id',
'actor_id', 'fullname',
null, null, 'priority'
);
A full working example can be found below:
$crud->setTable('film');
$crud->setSubject('Film', 'Films');
// With the ability to order the results
$crud->setRelationNtoN(
'actors', 'film_actor',
'actor', 'film_id',
'actor_id', 'fullname',
null, null, 'priority'
);
// Without the ability to order the results
$crud->setRelationNtoN(
'categories', 'film_category',
'category', 'film_id',
'category_id', 'name'
);
$output = $crud->render();
The result of the above code is here. As you can see we now have two new fields (actors and categories) with multiple select functionality.